1.swing中常用容器
Swing中常见的三种窗口JFrame,JDialog,JPanel。
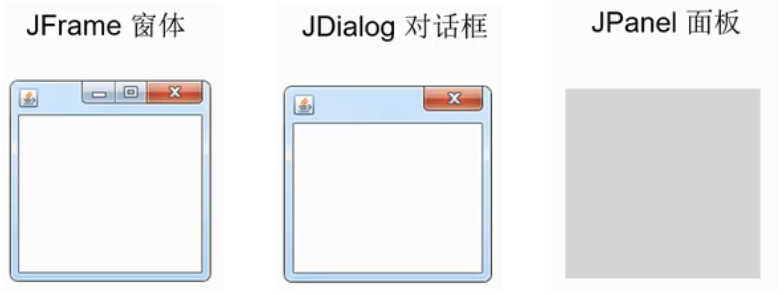
(1)JFrame
常见方法:
1.构造方法
// 创建窗体对象
JFrame f = new JFrame("窗体标题");
2.设置窗体可见:
// 设置窗体可见
f.setVisible(true);
3.设置点击窗体的执行的操作:
f.setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE);// DO_NOTHING_ON_CLOSE,不执行任何操作。
f.setDefaultCloseOperation(JFrame.HIDE_ON_CLOSE);// HIDE_ON_CLOSE,只隐藏界面,setVisible(false)。
f.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);// DISPOSE_ON_CLOSE,隐藏并释放窗体,dispose(),当最后一个窗口被释放后,则程序也随之运行结束。
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// EXIT_ON_CLOSE,直接关闭应用程序,System.exit(0)。一个main函数对应一整个程序
// 或者
this.setDefaultCloseOperation(0);// DO_NOTHING_ON_CLOSE,不执行任何操作。
this.setDefaultCloseOperation(1);// HIDE_ON_CLOSE,只隐藏界面,setVisible(false)。
this.setDefaultCloseOperation(2);// DISPOSE_ON_CLOSE,隐藏并释放窗体,dispose(),当最后一个窗口被释放后,则程序也随之运行结束。
this.setDefaultCloseOperation(3);// EXIT_ON_CLOSE,直接关闭应用程序,System.exit(0)。一个main函数对应一整个程序
4.设置窗体的大小和位置
// 设置完全可以大小,单位:像素
f.setSize(300,200);
// 设置坐标,单位:像素
f.setLocation(300,200);
// 设置窗体坐标和大小
f.setBounds(200,200,300,200);
// 让窗体显示在屏幕中心(无论窗体多大)
f.setLocationRelativeTo(null);
5.获取窗体容器:
// 获取窗体窗口
Container c = f.getContentPane();
JLabel l = new JLabel("这是一个窗体");
在容器中添加和删除组件:
JButton button_sure = new JButton("确定");
// 添加组件
c.add(button_sure);
// 删除组件
c.remove(1); // index
c.remove(button_sure);
做完添加和删除之后最好刷新容器:
// 验证容器中的组件
c.validate();
6.设置窗体是否可以改变大小:
// 设置窗体是否可以改变大小
f.setResizable(false);
7.设置窗体标题:
// 设置窗体标题
f.setTitle("窗体标题");
8.获取窗体的横纵坐标
// 获取横坐标
f.getX();
// 获取纵坐标
f.getY();
注意:在实际使用JFrame的时候,都是用自定义类来继承JFrame,然后在自定义类的构造方法中对窗体进行初始化.
(2)JDialog
常用方法与上面类似
示例代码:保证弹出对话框后阻塞父窗体
package Test;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Test extends JDialog {
public Test(JFrame frame) {
/*
* 第一个参数:父窗体对象
* 第二个参数:对话框标题
* 第三个参数:是否阻塞父窗体
*/
super(frame, "对话框", true);
// 获取对话框窗体容器
Container c = getContentPane();
// 添加一个对话框
c.add(new JLabel("这是一个对话框"));
// 设置对话框窗体的坐标和大小
setBounds(100, 100, 100, 100);
}
public static void main(String[] args) {
// 创建一个窗体
JFrame f = new JFrame("父窗体");
// 设置窗体的坐标和大小
f.setBounds(50, 50, 300, 300);
// 获取窗体f的容器
Container c = f.getContentPane();
// 创建一个按钮
JButton btn = new JButton("弹出对话框");
// 去除焦点框
btn.setFocusPainted(false);
// 使用流布局设置布局
c.setLayout(new FlowLayout());
// 将按钮添加到窗体中
c.add(btn);
// 设置窗体可见
f.setVisible(true);
// 设置窗体点击x号关闭程序
f.setDefaultCloseOperation(EXIT_ON_CLOSE);
// 对按钮添加监听事件
btn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Test dialog = new Test(f);
dialog.setVisible(true);
}
});
}
}
2.Swing中常用组件
Swing中常用组件有:JButton(按钮),JLabel(标签),JCheckBox(多选框),JRadioButton(单选按钮),JTextField(文本框),JPassword(密码框),JComBox(下拉框),JTextArea(文本框),JList(列表框),JOptionPane(对话框)等
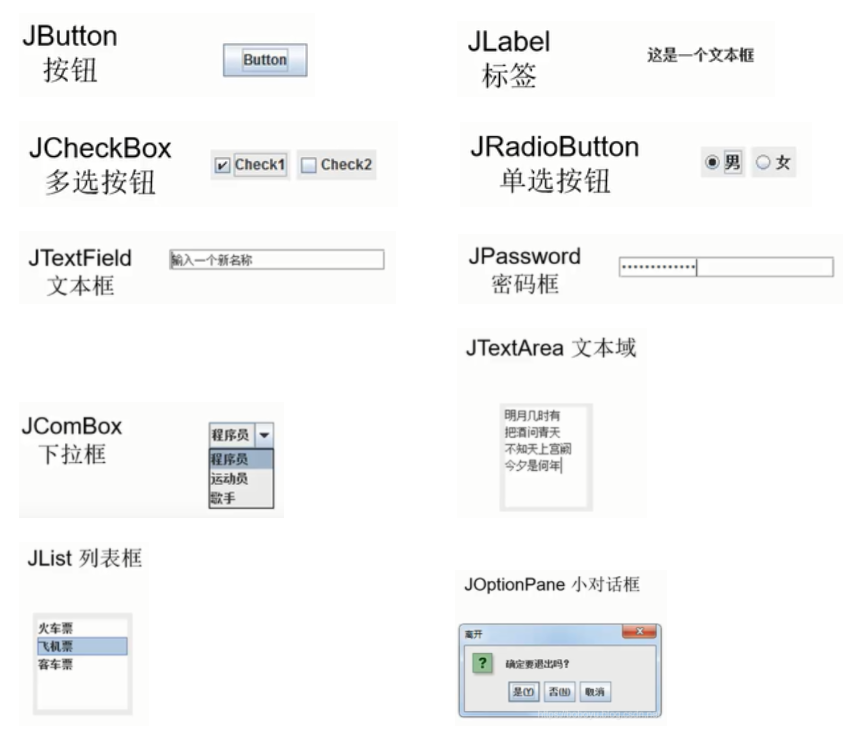
(1)JLabel
作用:在窗体上添加文字或图片
常用方法:
JLabel jLabel = new JLabel("JLabel组件");
// 设置字体(Font font)
jLabel.setFont(new Font("微软雅黑",Font.PLAIN,18));
// 设置图标(Icon icon)
jLabel.setIcon(new ImageIcon("image/qq.png"));
// 设置鼠标指针形状(Cursor cursor)
jLabel.setCursor(new Cursor(Cursor.HAND_CURSOR));
// 设置Tip文本(String text)
jLabel.setToolTipText("Tip文本");
// 设置大小(int width,int height)
jLabel.setSize(80,40);
// 设置位置(int x,int y)
jLabel.setLocation(50,40);
// ------JLabel的构造方法------
// 设置标签上的文字(String text)
jLabel.setText("JLabel文字");
// 获取标签上的文字
jLabel.getText();
// 设置背景颜色(Color bg)
jLabel.setBackground(Color.RED);
// 设置透明度(boolean isOpaque)
jLabel.setOpaque(true);
// 设置文字颜色(前景色)(Color fg),设置标签前景色,必须同时设置标签透明度!
jLabel.setForeground(Color.BLUE);
// 标签通常起提示作用,很少给标签添加监听器
示例:
import java.awt.Container;
import java.awt.Cursor;
import java.awt.Font;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class MyJFrame extends JFrame {
//创建标签的引用
private JLabel label01;
private JLabel label02;
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init()
{
//设置窗体
this.setSize(600,400);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("JLabel组件");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
Container container=this.getContentPane();
container.setLayout(null);
label01 = new JLabel("你好");
label01.setSize(200,100);
label01.setLocation(0,0);
//字体的大小:以磅作为单位
//setFont是很多组件都有的
label01.setFont(new Font("微软雅黑", Font.BOLD, 40));
label02 = new JLabel();
label02.setSize(300,300);
label02.setLocation(220,0);
//在标签中添加图片
//图片本身的像素大小 > 标签的大小:只显示图片的一部分
//在实际开发中,我们会首先设计好界面,然后然后确定好图片的大小,用制图软件去改变图片的像素大小
label02.setIcon(new ImageIcon("前进.png"));
//为鼠标进入标签区域变换形状
//Cursor类表示鼠标的各种形状,可以通过Cursor类的静态属性当作参数指定形状
label02.setCursor(new Cursor(Cursor.HAND_CURSOR));
//为标签添加tip提示框:即鼠标放在标签上,就会显示提示框
label02.setToolTipText("这是一个Tip");
container.add(label01);
container.add(label02);
}
public static void main(String[] args) {
MyJFrame frame =new MyJFrame();
frame.setVisible(true);
}
}
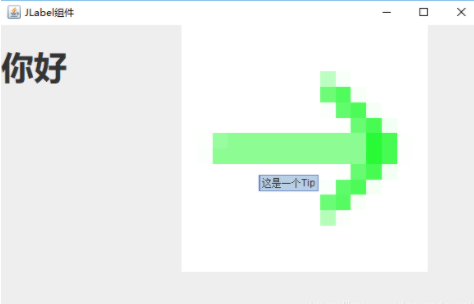
(2)文本框JTextField和密码框JPasswordField
作用:输入单行文本或密码
常用方法:
JTextField jTextField = new JTextField();
// 设置大小(int width,int height)
jTextField.setSize(80,40);
// 设置位置(int x,int y)
jTextField.setLocation(50,40);
// ------jTextField的构造方法------
// 设置文本框里的文字(String text)
jTextField.setText("jTextField文字");
// 获取文本框上的文字
jTextField.getText();
// 设置背景颜色(Color bg)
jTextField.setBackground(Color.RED);
// 设置透明度(boolean isOpaque)
jTextField.setOpaque(true);
// 设置文字颜色(前景色)(Color fg)
jTextField.setForeground(Color.BLUE);
JPasswordField jPasswordField = new JPasswordField();
// 设置大小(int width,int height)
jPasswordField.setSize(80,40);
// 设置位置(int x,int y)
jPasswordField.setLocation(50,40);
// ------jPasswordField的构造方法------
// 设置密码框上的文字(String text),一般不设置
jPasswordField.setText("jPasswordField文字");
// 获取密码框上的文字
jPasswordField.getPassword();
// 设置背景颜色(Color bg)
jPasswordField.setBackground(Color.RED);
// 设置透明度(boolean isOpaque)
jPasswordField.setOpaque(true);
// 设置文字颜色(前景色)(Color fg)
jPasswordField.setForeground(Color.BLUE);
jPasswordField.setEchoChar('+'); // 显示为+
文本框和密码框组件的ActionListener是用来处理按下回车事件的。
调用按钮组件的doClick方法相当于点了这个按钮。
获取密码框内容是jPasswordField.getPassword();
设置密码框回显字符是jPasswordField.setEchoChar(‘*’);
文本框的setColumns()方法在空布局的情况下才能够看到效果。
示例:
import java.awt.Container;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class MyJFrame extends JFrame {
//创建标签的引用
private JLabel userName_Label;
private JLabel password_Label;
private JTextField userName_TextField;
private JPasswordField passwordField;
private JButton login_Button;
private JButton cancel_Button;
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init() {
//设置窗体
this.setSize(600, 400);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("JLabel组件");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
Container container = this.getContentPane();
container.setLayout(null);
//对6个组件进行实例化
userName_Label = new JLabel("用户名");
password_Label = new JLabel("密码");
userName_TextField = new JTextField();
passwordField = new JPasswordField();
login_Button = new JButton("登录");
cancel_Button = new JButton("取消");
//设置用户名标签的大小、位置、字体
userName_Label.setSize(70, 40);
userName_Label.setLocation(100, 60);
userName_Label.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//设置密码标签的大小、位置、字体
password_Label.setSize(70, 40);
password_Label.setLocation(100, 160);
password_Label.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//设置用户名输入框的大小、位置
userName_TextField.setSize(300, 40);
userName_TextField.setLocation(200, 60);
//设置文本输入框中的限制字符的个数,该方法对null布局不起作用
//只能输入5个字符(在非null布局下),但是每个字符的宽度不一样,该方法,以字符m为标准限制字符个数
userName_TextField.setColumns(5);
//实现在用户名输入框中按下回车键时,光标切换到密码输入框中
userName_TextField.addActionListener(new ActionListener() { //文本框中使用频率最高的事件是按下回车键
@Override
public void actionPerformed(ActionEvent e) {
//将光标切换到密码框当中
passwordField.requestFocus();
}
});
//设置密码输入框的大小、位置
passwordField.setSize(300, 40);
passwordField.setLocation(200, 160);
//设置密码输入框中的回显字符,即在密码框中显示的字符
passwordField.setEchoChar('*');
//实现在密码输入框中按下回车后直接登录
passwordField.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
login_Button.doClick(); //点击按钮
}
});
//设置登录按钮的大小和位置
login_Button.setSize(150, 40);
login_Button.setLocation(130, 240);
login_Button.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//模拟登录
login_Button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String userName = userName_TextField.getText();
String password = new String(passwordField.getPassword());
System.out.println("用户名:" + userName);
System.out.println("密码:" + password);
System.out.println("登录中。。。");
}
});
//设置取消按钮的大小和位置
cancel_Button.setSize(150, 40);
cancel_Button.setLocation(320, 240);
cancel_Button.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//将6个组件添加到界面容器当中
container.add(userName_Label);
container.add(password_Label);
container.add(passwordField);
container.add(userName_TextField);
container.add(login_Button);
container.add(cancel_Button);
}
public static void main(String[] args) {
MyJFrame frame = new MyJFrame();
frame.setVisible(true);
}
}
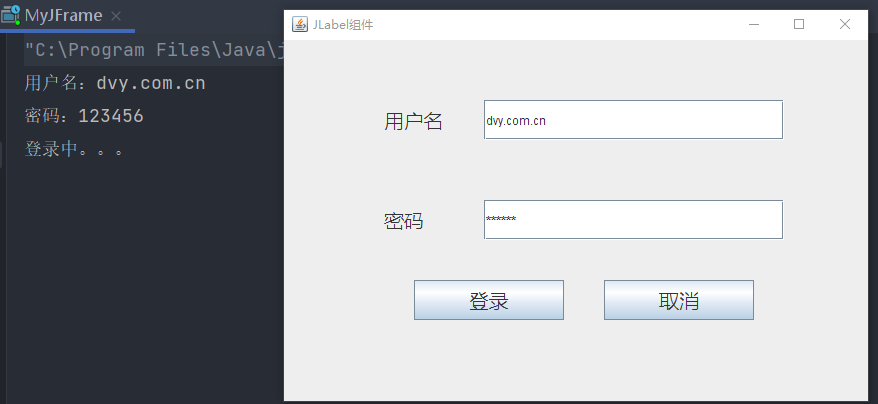
(3)文本区JTextArea
方法:
JTextArea jTextArea = new JTextArea();
// 设置大小(int width,int height)
jTextArea.setSize(600, 300);
// 设置位置(int x,int y)
jTextArea.setLocation(0, 50);
// 设置边距
jTextArea.setMargin(new Insets(5, 5, 5, 5));
// ------jTextArea的构造方法------
// 设置文本框里的文字(String text)
jTextArea.setText("jTextArea文字");
// 获取文本框上的文字
jTextArea.getText();
// 设置背景颜色(Color bg)
jTextArea.setBackground(Color.LIGHT_GRAY);
// 设置透明度(boolean isOpaque)
jTextArea.setOpaque(true);
// 设置文字颜色(前景色)(Color fg)
jTextArea.setForeground(Color.BLUE);
示例
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class MyJFrame extends JFrame {
//创建标签的引用
private JLabel userName_Label;
private JLabel password_Label;
private JTextField userName_TextField;
private JPasswordField passwordField;
private JButton login_Button;
private JButton cancel_Button;
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init() {
//设置窗体
this.setSize(400, 300);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("JLabel组件");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
Container container = this.getContentPane();
container.setLayout(null);
JLabel jLabel = new JLabel("这是文本框展示");
jLabel.setSize(300, 40);
JTextArea jTextArea = new JTextArea();
// 设置大小(int width,int height)
jTextArea.setSize(400, 260);
// 设置位置(int x,int y)
jTextArea.setLocation(0, 50);
// 设置边距
jTextArea.setMargin(new Insets(5, 5, 5, 5));
// ------jTextArea的构造方法------
// 设置文本框里的文字(String text)
jTextArea.setText("jTextArea文字");
// 获取文本框上的文字
jTextArea.getText();
// 设置背景颜色(Color bg)
jTextArea.setBackground(Color.LIGHT_GRAY);
// 设置透明度(boolean isOpaque)
jTextArea.setOpaque(true);
// 设置文字颜色(前景色)(Color fg)
jTextArea.setForeground(Color.BLUE);
//将组件添加到界面容器当中
container.add(jLabel);
container.add(jTextArea);
}
public static void main(String[] args) {
MyJFrame frame = new MyJFrame();
frame.setVisible(true);
}
}
(4)复选框JCheckBox
复选框,同时也是选项框。
复选框和按钮有一个共同的祖先:AbstractButton
复选框当作按钮:用ActionListener来处理点击事件。
复选框当作选项:用ItemListener来处理选项状态变化事件。
设置复选框选中状态:setSelected(boolean b)
获取选中状态:isSelected()
常用方法略:基本与其它组件上同
复选框本身就是一个按钮,只要点在按钮区域点击哪里都是一样的,都会被选中
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import javax.swing.*;
public class MyJFrame extends JFrame {
//作用一:检查框
private JCheckBox confirm_CheckBox;
private JButton next_Button;
//作用二:
private String[] city_names;
private JCheckBox[] cities_CheckBoxs;
private JButton ok_Button;
private JLabel cities_Label;
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init() {
//设置窗体
this.setSize(500, 400);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("复选框演示");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
Container container = this.getContentPane();
container.setLayout(null);
JLabel jl1 = new JLabel("作用一:实现确认");
//设置按钮的大小、位置、字体、不可见
jl1.setSize(400, 40);
jl1.setLocation(30, 0);
jl1.setFont(new Font("微软雅黑", Font.BOLD, 20));
JLabel jl2 = new JLabel("作用二:实现多选");
//设置按钮的大小、位置、字体、不可见
jl2.setSize(400, 40);
jl2.setLocation(30, 170);
jl2.setFont(new Font("微软雅黑", Font.BOLD, 20));
JLabel jl3 = new JLabel("www.dvy.com.cn");
//设置按钮的大小、位置、字体、不可见
jl3.setSize(300, 40);
jl3.setLocation(150, 300);
jl2.setFont(new Font("微软雅黑", Font.BOLD, 22));
jl3.setOpaque(true);
jl3.setBackground(new Color(1, 152, 184));
jl3.setForeground(Color.WHITE);
//jl3.setHorizontalAlignment(SwingConstants.CENTER);
jl3.setHorizontalAlignment(SwingConstants.RIGHT);
confirm_CheckBox = new JCheckBox("我已经阅读并接受条款");
// 去掉文字外的边框
confirm_CheckBox.setFocusPainted(false);
next_Button = new JButton("下一步");
next_Button.setFocusPainted(false);
cities_Label = new JLabel();
city_names = new String[]{"北京", "上海", "广州", "深圳"};
cities_CheckBoxs = new JCheckBox[city_names.length];
ok_Button = new JButton("提交");
ok_Button.setFocusPainted(false);
//设置复选框的大小、位置、字体
confirm_CheckBox.setSize(250, 40);
confirm_CheckBox.setLocation(30, 30);
confirm_CheckBox.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//设置复选框选中按钮为可用状态,复选框未被选中为不可用状态
confirm_CheckBox.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
//复选框是否被选中
boolean flag = confirm_CheckBox.isSelected();
next_Button.setEnabled(flag);
if (!flag) {
int option = JOptionPane.showConfirmDialog(null, "是否同意条款?", "提示:", JOptionPane.YES_NO_OPTION);
// 设置选项框选中状态
confirm_CheckBox.setSelected(option == JOptionPane.YES_OPTION);
// 设置按钮的禁用状态
next_Button.setEnabled(option == JOptionPane.YES_OPTION);
if (option == JOptionPane.NO_OPTION) {
System.out.println("你未同意条款");
} else if (option == JOptionPane.YES_OPTION) {
System.out.println("已同意条款");
}
} else {
System.out.println("已同意条款");
}
}
});
confirm_CheckBox.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
//复选框是否被选中
boolean flag = confirm_CheckBox.isSelected();
next_Button.setEnabled(flag);
}
});
//设置按钮的大小、位置、字体、不可见
next_Button.setSize(100, 40);
next_Button.setLocation(30, 80);
next_Button.setFont(new Font("微软雅黑", Font.PLAIN, 20));
next_Button.setEnabled(false);
//设置标签
cities_Label.setSize(200, 40);
cities_Label.setLocation(30, 150);
cities_Label.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//设置复选框
for (int i = 0; i < cities_CheckBoxs.length; i++) {
cities_CheckBoxs[i] = new JCheckBox(city_names[i]);
cities_CheckBoxs[i].setSize(70, 40);
cities_CheckBoxs[i].setLocation(30 + i * 70, 200);
cities_CheckBoxs[i].setFont(new Font("微软雅黑", Font.PLAIN, 18));
cities_CheckBoxs[i].setFocusPainted(false);
container.add(cities_CheckBoxs[i]);
}
//设置ok按钮
ok_Button.setSize(80, 40);
ok_Button.setLocation(30, 250);
ok_Button.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//模拟提交
ok_Button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String string = new String();
for (int i = 0; i < cities_CheckBoxs.length; i++) {
if (cities_CheckBoxs[i].isSelected()) {
string = string + cities_CheckBoxs[i].getText() + " ";
}
}
if (!"".equals(string)) {
System.out.println("选中了:" + string);
} else {
System.out.println("请至少选中一项!");
}
System.out.println("www.dvy.com.cn");
}
});
//将组件添加到界面容器当中
container.add(jl1);
container.add(confirm_CheckBox);
container.add(next_Button);
container.add(jl2);
container.add(cities_Label);
container.add(ok_Button);
container.add(jl3);
}
public static void main(String[] args) {
MyJFrame frame = new MyJFrame();
frame.setVisible(true);
}
}
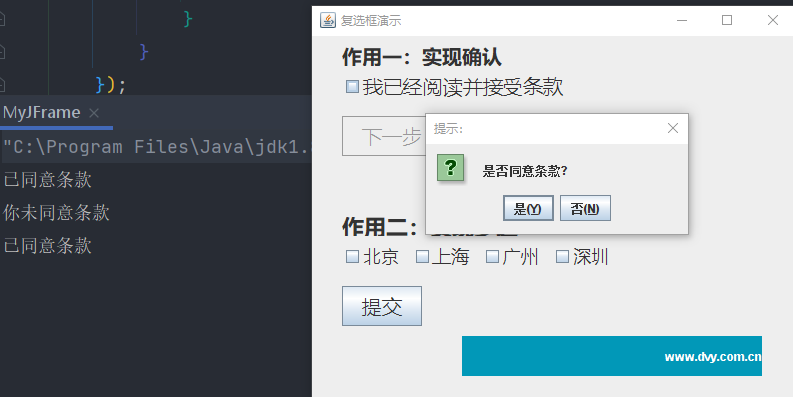
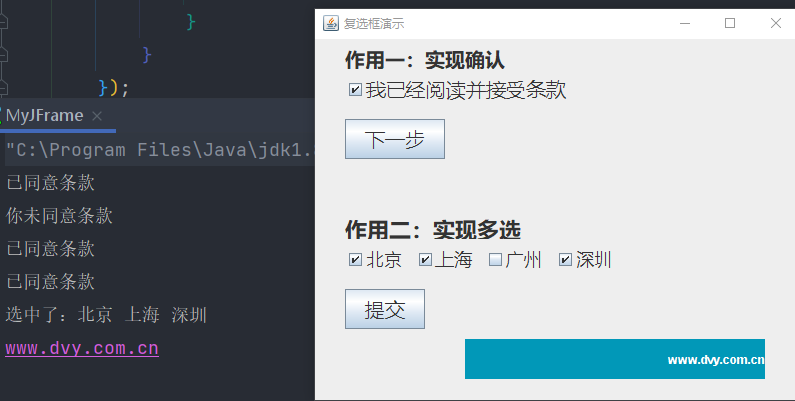
(5)单选按钮组件
示例:
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
import javax.swing.border.MatteBorder;
public class MyJFrame extends JFrame implements ActionListener {
JTextField xuehao;
JTextField xingming;
JTextField banji;
JTextField xueyuan;
JPanel buttonPanel, sexPanel;
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init() {
//设置窗体
this.setSize(260, 360);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("单选框演示");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
JLabel label1 = new JLabel(" 添加基本信息", SwingConstants.CENTER);
label1.setHorizontalAlignment(SwingConstants.CENTER);
label1.setSize(260, 40);
label1.setFont(new Font("微软雅黑", Font.BOLD, 20));
MatteBorder matteBorder = new MatteBorder(0, 0, 1, 0, Color.GRAY);
label1.setBorder(matteBorder);
JLabel label2 = new JLabel("学号:", SwingConstants.CENTER);
label2.setSize(100, 20);
label2.setLocation(5, 60);
xuehao = new JTextField("", 30);
xuehao.setSize(100, 30);
xuehao.setLocation(88, 55);
JLabel label3 = new JLabel("姓名:", SwingConstants.CENTER);
label3.setSize(100, 20);
label3.setLocation(5, 100);
xingming = new JTextField("", 30);
xingming.setSize(100, 30);
xingming.setLocation(88, 95);
JLabel label4 = new JLabel("性别:", SwingConstants.CENTER);
label4.setSize(100, 20);
label4.setLocation(5, 140);
// 设置单选框
JRadioButton sex1 = new JRadioButton("男");
JRadioButton sex2 = new JRadioButton("女");
sex1.setFocusPainted(false);
sex2.setFocusPainted(false);
sexPanel = new JPanel();
sexPanel.setToolTipText("请选择性别!");
sexPanel.setSize(260, 30);
sexPanel.setBackground(new Color(230, 230, 230));
sexPanel.setLocation(0, 132);
sexPanel.add(sex1);
sexPanel.add(sex2);
ButtonGroup group = new ButtonGroup();
group.add(sex1);
group.add(sex2);
JLabel label5 = new JLabel("班级:", SwingConstants.CENTER);
label5.setSize(100, 20);
label5.setLocation(5, 180);
banji = new JTextField("", 30);
banji.setSize(100, 30);
banji.setLocation(88, 175);
JLabel label6 = new JLabel("学院:", SwingConstants.CENTER);
label6.setSize(100, 20);
label6.setLocation(5, 220);
xueyuan = new JTextField("", 30);
xueyuan.setSize(100, 30);
xueyuan.setLocation(88, 215);
JButton button1 = new JButton("添加");
button1.setFocusPainted(false);
JButton button2 = new JButton("重置");
button2.setFocusPainted(false);
buttonPanel = new JPanel();
buttonPanel.setSize(260, 50);
buttonPanel.setLocation(0, 260);
buttonPanel.add(button1);
button1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String xh = xuehao.getText();
String xm = xingming.getText();
String xb = "";
String bj = banji.getText();
String xy = xueyuan.getText();
String all = "";
for (Component c : sexPanel.getComponents()) {
if (c instanceof JRadioButton) {
if (((JRadioButton) c).isSelected()) {
xb += ((JRadioButton) c).getText();
}
}
}
all = "学号:" + xh + "\n" + "姓名:" + xm + "\n" + "性别:" + xb + "\n" + "班级:" + bj + "\n" + "学院:" + xy + "\n";
javax.swing.JOptionPane.showMessageDialog(null, all);
}
});
buttonPanel.add(button2);
button2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
}
});
Container container = this.getContentPane();
container.setLayout(null);
// 标题
container.add(label1);
container.add(label2);
container.add(xuehao);
container.add(label3);
container.add(xingming);
container.add(label4);
container.add(sexPanel);
container.add(label5);
container.add(xueyuan);
container.add(label6);
container.add(banji);
container.add(buttonPanel);
}
public void actionPerformed(ActionEvent arg) {
}
public static void main(String[] args) {
MyJFrame frame = new MyJFrame();
frame.setVisible(true);
}
}
效果:
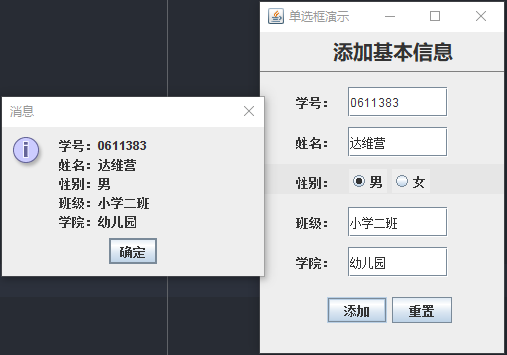
(6)进度条组件
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class MyJFrame extends JFrame implements ActionListener {
static Thread thread;
JProgressBar progressBar;
private int count;
JLabel jLabel;
Container container;
MyJFrame myJFrame;
public MyJFrame() {
progressBar = new JProgressBar();
progressBar.setStringPainted(true);
container = getContentPane();
container.add(progressBar, BorderLayout.NORTH);//在不指定布局管理器的情况下,默认使用BorderLayout。 若不使用布局管理器,需明确说明setLayout(null)
this.setTitle("Swing进度条");
this.setVisible(true);
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
myJFrame = this;
this.creatThread();
thread.start();
}
@Override
public void actionPerformed(ActionEvent e) {
}
class Thread_instance implements Runnable {
boolean isContinue = true;
public void setContinue(boolean b) {
this.isContinue = b;
}
@Override
public void run() {
// TODO Auto-generated method stub
count = 0;
while (true) {
progressBar.setValue(++count);
if (count % 5 == 0) {
try {
jLabel = new JLabel("请等待3秒钟");
myJFrame.setTitle("请等待3秒!");
Thread.sleep(3000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
System.out.println("当前程序被中断");
break;
}
} else {
myJFrame.setTitle("Swing进度条");
try {
Thread.sleep(1000);
if (!isContinue) {
break;
}
} catch (InterruptedException e) {
// TODO Auto-generated catch block
System.out.println("当前程序被中断");
break;
}
}
}
}
}
void creatThread() {
thread = new Thread(new Thread_instance());
}
static void init(JFrame frame, int width, int height) {
frame.setSize(width, height);
}
public static void main(String[] args) {
init(new MyJFrame(), 300, 200);
}
}
效果:
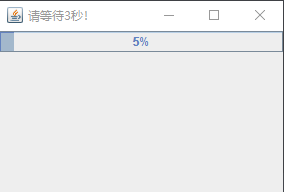
(7)列表框组件JList
1)创建列表框
列表框只是展示数据的窗口,我们看到的列表框的数据并不是来自于列表本身,这些数据来自于它所创建的数据容器
有三种可以传递给列表的数据容器
第一选择:ListModel(接口):我们可以定义它的实现类DefaultListModel传递给JList的构造方法
第二选择:Vector
第三选择:数组
import java.awt.Color;
import java.awt.Container;
import java.awt.Font;
import java.util.Vector;
import javax.swing.BorderFactory;
import javax.swing.DefaultListModel;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
public class MyJFrame extends JFrame {
private JLabel select_Label;
private JList<String> left_List;
//String[] leftData;
//Vector<String> leftData;
DefaultListModel<String> leftData;
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init() {
//设置窗体
this.setSize(600, 400);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("创建列表框演示");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
Container container = this.getContentPane();
container.setLayout(null);
//要传递给JList的数据容器
//leftData = new String[]{"关羽","赵云","黄忠","马超","魏延","张飞"};
//leftData =new Vector<String>(); //Vector并不能像数组一次性添加数据,必须通过add方法添加
//leftData.add("孙悟空");
//leftData.add("猪八戒");
//leftData.add("沙僧");
leftData = new DefaultListModel<String>();
leftData.addElement("孙悟空");
leftData.addElement("猪八戒");
leftData.addElement("沙僧");
//对组件进行实例化
select_Label = new JLabel("你准备带谁出征?");
//left_List = new JList<String>(leftData);
//left_List = new JList<String>();
//left_List.setListData(leftData);
//left_List = new JList<String>(leftData);
left_List = new JList<String>();
left_List.setModel(leftData);
//设置标签
select_Label.setSize(200, 40);
select_Label.setLocation(30, 30);
select_Label.setFont(new Font("微软雅黑", Font.PLAIN, 20));
//设置列表
left_List.setSize(200, 240);
left_List.setLocation(30, 80);
left_List.setFont(new Font("微软雅黑", Font.PLAIN, 20));
left_List.setBorder(BorderFactory.createLineBorder(Color.GRAY, 1));
container.add(select_Label);
container.add(left_List);
}
public static void main(String[] args) {
MyJFrame frame = new MyJFrame();
frame.setVisible(true);
}
}
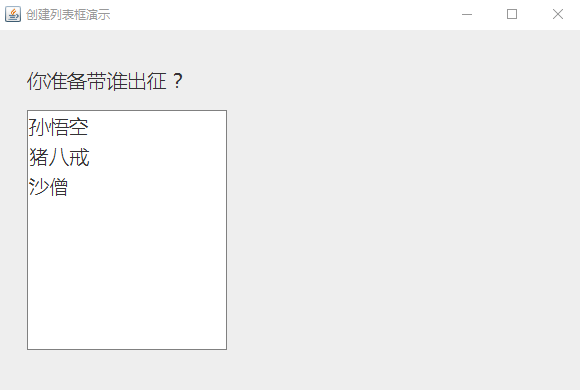
(8)树形组件JTree
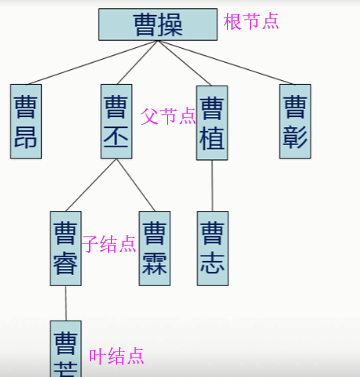
创建树形组件,只能是先添加根结点,然后再逐层去添加子节点
1)硬编码创建树形组件
此种方式用户不能新增,删除任何结点
import java.awt.Container;
import java.awt.Font;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTree;
import javax.swing.tree.DefaultMutableTreeNode;
public class MyJFrame extends JFrame {
private JTree familyTree;
private JScrollPane family_JScrollPane;
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init()
{
//设置窗体
this.setSize(600,400);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("创建列表框演示");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
Container container=this.getContentPane();
container.setLayout(null);
//创建一个根节点
DefaultMutableTreeNode root = new DefaultMutableTreeNode("曹操");
DefaultMutableTreeNode a1 = new DefaultMutableTreeNode("曹昂");
DefaultMutableTreeNode a2 = new DefaultMutableTreeNode("曹丕");
DefaultMutableTreeNode a3 = new DefaultMutableTreeNode("曹植");
DefaultMutableTreeNode a4 = new DefaultMutableTreeNode("曹彰");
root.add(a1);
root.add(a2);
root.add(a3);
root.add(a4);
DefaultMutableTreeNode b1 = new DefaultMutableTreeNode("曹睿");
DefaultMutableTreeNode b2 = new DefaultMutableTreeNode("曹霖");
DefaultMutableTreeNode b3 = new DefaultMutableTreeNode("曹志");
a2.add(b1);
a2.add(b2);
a3.add(b3);
DefaultMutableTreeNode c1 = new DefaultMutableTreeNode("曹芳");
b1.add(c1);
//创建树形组件
familyTree = new JTree(root);
familyTree.setFont(new Font("微软雅黑",Font.PLAIN,20));
//创建滚动面板
family_JScrollPane = new JScrollPane(familyTree);
family_JScrollPane.setSize(300,250);
family_JScrollPane.setLocation(30, 30);
container.add(family_JScrollPane);
}
public static void main(String[] args) {
MyJFrame frame =new MyJFrame();
frame.setVisible(true);
}
}
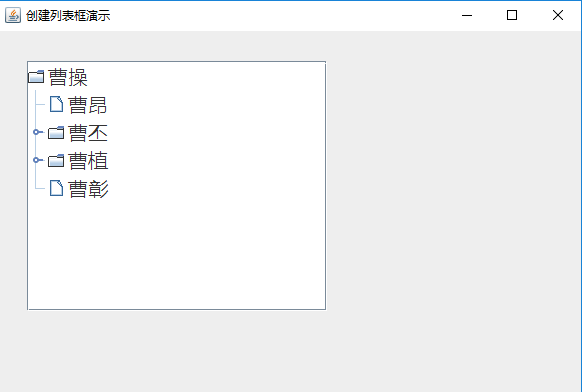
2)动态创建树形组件
常用方法:
树组件自身没有滚动条,需放入JScrollPane中展现全部数据设置字体--setFont(Font font)
调用构造方法JTree()所创建的树并非空树,而是具有默认节点树节点类--DefaultMutableTreeNode
构造方法--DefaultMutableTreeNode(Object userObject添加子节点--add(MutableTreeNode newChild)重命名节点--setUserObject(Object userObject)判断节点是否为根节点--isRoot()脱离父节点--removeFromParent)
设置单选模式--setSelectionMode(int mode)
展开树上某条路径上的节点--expandPath(TreePath path)更新组件界面--updateUI()
通过TreeSelectionListener监听器处理用户选择节点事件
研究构造方法JTree()添加默认节点的过程与细节获得子节点数量--getChildCount()得到所有的子节点--children()
移除子节点--remove(MutableTreeNode aChild)移除子节点--remove(int childIndex)
获得指定索引子节点--getChildAt(int index)
获得第一/最后子节点--getFirstChild(/getLastChild()
获得前一个/后一个节点--getPreviousNode()/getNextNode()
示例:
import java.awt.Container;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextField;
import javax.swing.JTree;
import javax.swing.event.TreeSelectionEvent;
import javax.swing.event.TreeSelectionListener;
import javax.swing.tree.DefaultMutableTreeNode;
import javax.swing.tree.TreePath;
import javax.swing.tree.TreeSelectionModel;
import javax.xml.transform.Templates;
public class MyJFrame extends JFrame {
private JTree dynTree;
private JScrollPane dyn_JScrollPane;
private DefaultMutableTreeNode rooTreeNode;
private DefaultMutableTreeNode selectedNode;
private JTextField nodeName_TextField;
private JButton createButton;
private JButton renameButton;
private JButton deleteButton;
private TreePath path; //用户选择的结点到根结点的路径
//在构造界面的时候就进行初始化界面
public MyJFrame() {
init();
}
//初始化界面
private void init() {
//设置窗体
this.setSize(600, 400);//设置窗体大小
this.setLocationRelativeTo(null);//设置窗体居中显示
this.setTitle("创建列表框演示");//设置窗体标题
this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置点击窗体x号时退出程序
Container container = this.getContentPane();
container.setLayout(null);
//实例化组件
rooTreeNode = new DefaultMutableTreeNode("根节点"); //创建根节点
dynTree = new JTree(rooTreeNode); //创建一颗以rootTreeNode为根节点的树形组件
dyn_JScrollPane = new JScrollPane(dynTree);
createButton = new JButton("新增");
deleteButton = new JButton("删除");
renameButton = new JButton("修改");
nodeName_TextField = new JTextField();
//设置树形组件
dynTree.setFont(new Font("微软雅黑", Font.PLAIN, 18));
//设置树形组件的单选模式,只能同时被选中一个结点
//先获得树形组件的选择模型对象,然后选择对象模型的单选模式
dynTree.getSelectionModel().setSelectionMode(TreeSelectionModel.SINGLE_TREE_SELECTION);
//为树形组件添加选中结点的监听器
dynTree.addTreeSelectionListener(new TreeSelectionListener() {
//一旦用户选中结点的状态发生变化,立刻执行此方法
//用户必须两次选择的结点不同才会起作用,因为这样才代表结点状态改变
@Override
public void valueChanged(TreeSelectionEvent e) {
//当我们从树形组件上选择了一条结点的时候,那么从根结点到它的路径是确定的(因为是单继承)
path = e.getPath(); //获得根节点到被选中的结点的唯一路径
}
});
//设置滚动面板
dyn_JScrollPane.setSize(350, 250);
dyn_JScrollPane.setLocation(200, 30);
dyn_JScrollPane.setFont(new Font("微软雅黑", Font.PLAIN, 18));
//设置文本框
nodeName_TextField.setSize(80, 30);
nodeName_TextField.setLocation(290, 300);
nodeName_TextField.setFont(new Font("微软雅黑", Font.PLAIN, 18));
//设置新增按钮
createButton.setSize(80, 30);
createButton.setLocation(290, 300);
createButton.setFont(new Font("微软雅黑", Font.PLAIN, 18));
createButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
//获得树形组件中被选中的结点
//这个方法的返回值是Object类型,此处需要强制类型转换
selectedNode = (DefaultMutableTreeNode) dynTree.getLastSelectedPathComponent();
String nameString = nodeName_TextField.getText();
if (checkNode_or_checkInput(selectedNode, nameString)) {
selectedNode.add(new DefaultMutableTreeNode(nameString));
//展开被选中结点的路径上的所有结点
dynTree.expandPath(path);
//当我们给树形组件新增一个结点的时候,树形图上不会立刻显示新增的结点
//调用以下方法刷新树形组件
dynTree.updateUI();
//创建之后清空文本域
nodeName_TextField.setText("");
}
}
});
//设置删除按钮
deleteButton.setSize(80, 30);
deleteButton.setLocation(380, 300);
deleteButton.setFont(new Font("微软雅黑", Font.PLAIN, 18));
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
selectedNode = (DefaultMutableTreeNode) dynTree.getLastSelectedPathComponent();
if (selectedNode == null) {
System.out.println("请选中一个结点");
return;
}
if (selectedNode.isRoot()) {
System.out.println("不能删除根节点哦");
return;
}
selectedNode.removeFromParent();
dynTree.updateUI();
}
});
//设置修改按钮
renameButton.setSize(80, 30);
renameButton.setLocation(470, 300);
renameButton.setFont(new Font("微软雅黑", Font.PLAIN, 18));
renameButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
//获得树形组件中被选中的结点
//这个方法的返回值是Object类型,此处需要强制类型转换
selectedNode = (DefaultMutableTreeNode) dynTree.getLastSelectedPathComponent();
String nameString = nodeName_TextField.getText();
//不将上述两行代码进行封装的原因是因为上面两行是采集数据,而checkNode_or_checkInput是验证数据
//从逻辑上来说,我们不应该把采集数据和验证数据放在一个功能中
if (checkNode_or_checkInput(selectedNode, nameString)) {
selectedNode.setUserObject(nameString);
dynTree.updateUI();
//修改之后清空文本域
nodeName_TextField.setText("");
}
}
});
//设置文本域
nodeName_TextField.setSize(80, 30);
nodeName_TextField.setLocation(200, 300);
nodeName_TextField.setFont(new Font("微软雅黑", Font.PLAIN, 18));
//将组件添加到容器中
container.add(dyn_JScrollPane);
container.add(createButton);
container.add(deleteButton);
container.add(renameButton);
container.add(nodeName_TextField);
}
public static void main(String[] args) {
MyJFrame frame = new MyJFrame();
frame.setVisible(true);
}
//检验用户是否选择了结点或进行了输入
private boolean checkNode_or_checkInput(DefaultMutableTreeNode node, String name) {
//判断用户是否选择了一个结点
if (node == null) {
System.out.println("请选择一个结点");
return false;
}
//判断用户是否进行了输入
if ("".equals(name)) {
System.out.println("请填写结点名称");
return false;
}
return true;
}
}
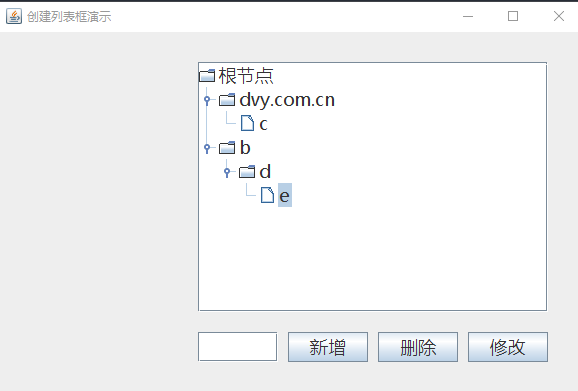